Introduction
In modern software development, APIs serve as the critical connectors between services, making reliable API testing essential for maintaining application quality. Recent studies show that teams spend an average of 25 hours per month debugging API-related issues, with 68% of these problems being discoverable through proper testing. This guide will show you how to leverage Playwright for efficient, reliable API testing that integrates seamlessly with your existing workflows.
Understanding API Testing Fundamentals
Before diving into Playwright, let’s establish what makes API testing effective:
API testing validates the business logic, data accuracy, and reliability of your application’s programming interfaces. Unlike UI testing, which focuses on user interactions, API testing ensures your application’s core functionality works correctly at the service level.
Core Components of API Testing
Functional Validation
- Request/response integrity
- Data processing accuracy
- Error handling
- Edge cases and boundary testing
Non-functional Testing
- Performance under load
- Security and authentication
- Data encryption
- Rate limiting and throttling
Why Playwright Stands Out for API Testing
Playwright has earned its reputation in browser automation, but its API testing capabilities offer unique advantages that set it apart:
Technical Advantages
- Request Interception: Modify and inspect network requests in real-time
- Parallel Test Execution: Run tests concurrently with intelligent request queuing
- Cross-Origin Support: Handle CORS and complex authentication scenarios effortlessly
- Built-in Assertions: Rich assertion library specifically designed for API testing
- TypeScript Support: First-class TypeScript support with excellent type definitions
Getting Started with Playwright
Installation and Setup
First, create a new project and install Playwright:
npm init -y
npm init playwright@latest
Configure your test environment by creating a playwright.config.ts
:
import { PlaywrightTestConfig } from '@playwright/test';
const config: PlaywrightTestConfig = {
timeout: 30000,
retries: 2,
use: {
baseURL: process.env.API_BASE_URL || 'http://localhost:3000',
extraHTTPHeaders: {
'Accept': 'application/json',
},
},
reporter: [
['html'],
['json', { outputFile: 'test-results/json-report.json' }]
],
};
export default config;
Writing Your First API Test
Create your first test file tests/api/basic.spec.ts
:
import { test, expect } from '@playwright/test';
test.describe('Basic API Testing', () => {
// Setup: Run before all tests in this describe block
test.beforeAll(async ({ request }) => {
// Initialize test data or authentication tokens
});
test('should successfully fetch user data', async ({ request }) => {
// Send GET request
const response = await request.get('/api/users/1');
// Validate response
expect(response.ok()).toBeTruthy();
expect(response.status()).toBe(200);
const userData = await response.json();
expect(userData).toHaveProperty('id');
expect(userData).toHaveProperty('email');
});
test('should handle invalid requests gracefully', async ({ request }) => {
// Test error handling
const response = await request.get('/api/users/invalid');
expect(response.status()).toBe(404);
const errorData = await response.json();
expect(errorData).toHaveProperty('error');
});
});
Advanced Testing Patterns
Authentication Handling
import { test as base } from '@playwright/test';
// Create a custom test fixture for authenticated requests
type AuthFixtures = {
authRequest: typeof base.request;
};
const test = base.extend<AuthFixtures>({
authRequest: async ({ request }, use) => {
const token = await getAuthToken(); // Your token acquisition logic
const authenticatedRequest = request.extend({
headers: {
'Authorization': `Bearer ${token}`,
},
});
await use(authenticatedRequest);
},
});
test('authenticated API call', async ({ authRequest }) => {
const response = await authRequest.get('/api/protected-resource');
expect(response.ok()).toBeTruthy();
});
Schema Validation
import { test, expect } from '@playwright/test';
import Ajv from 'ajv';
const ajv = new Ajv();
const userSchema = {
type: 'object',
required: ['id', 'email', 'name'],
properties: {
id: { type: 'number' },
email: { type: 'string', format: 'email' },
name: { type: 'string' },
role: { type: 'string', enum: ['user', 'admin'] }
}
};
test('validate user response schema', async ({ request }) => {
const response = await request.get('/api/users/1');
const userData = await response.json();
const validate = ajv.compile(userSchema);
const isValid = validate(userData);
expect(isValid).toBeTruthy();
if (!isValid) {
console.log('Validation errors:', validate.errors);
}
});
Performance Testing
Load Testing Example
import { test, expect } from '@playwright/test';
test('API performance under load', async ({ request }) => {
const startTime = Date.now();
const requests = Array(100).fill(null).map(() =>
request.get('/api/users')
);
const responses = await Promise.all(requests);
const endTime = Date.now();
// Analyze results
const totalTime = endTime - startTime;
const successfulRequests = responses.filter(r => r.ok()).length;
const avgResponseTime = totalTime / requests.length;
expect(successfulRequests).toBe(requests.length);
expect(avgResponseTime).toBeLessThan(1000); // 1 second threshold
});
Error Handling and Debugging
Common Patterns for Error Cases
test('handle various error scenarios', async ({ request }) => {
// Test rate limiting
const rapidRequests = Array(10).fill(null).map(() =>
request.get('/api/rate-limited-endpoint')
);
const responses = await Promise.all(rapidRequests);
// Verify rate limiting behavior
const rateLimited = responses.some(r => r.status() === 429);
expect(rateLimited).toBeTruthy();
// Test malformed requests
const malformedResponse = await request.post('/api/users', {
data: { invalid: 'data' }
});
expect(malformedResponse.status()).toBe(400);
// Verify error response structure
const errorData = await malformedResponse.json();
expect(errorData).toHaveProperty('message');
expect(errorData).toHaveProperty('code');
});
CI/CD Integration
GitHub Actions Example
name: API Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Install Playwright browsers
run: npx playwright install --with-deps
- name: Run API tests
run: npx playwright test
env:
API_BASE_URL: ${{ secrets.API_BASE_URL }}
API_TOKEN: ${{ secrets.API_TOKEN }}
- name: Upload test results
if: always()
uses: actions/upload-artifact@v3
with:
name: playwright-report
path: playwright-report/
Best Practices and Tips
Environment Management
- Use environment variables for configuration
- Maintain separate configs for different environments
- Never commit sensitive data to version control
Test Organization
- Group related tests using
test.describe
- Use fixtures for common setup
- Follow the Arrange-Act-Assert pattern
Error Handling
- Test both success and failure scenarios
- Validate error response structures
- Include timeout handling
Performance Considerations
- Run tests in parallel when possible
- Use test sharding for large test suites
- Monitor and log test execution times
Monitoring and Reporting
Playwright provides built-in reporters, but you can enhance them:
import { Reporter } from '@playwright/test/reporter';
class CustomReporter implements Reporter {
onTestBegin(test) {
console.log(`Starting test: ${test.title}`);
}
onTestEnd(test, result) {
console.log(`Test ${test.title} finished with status: ${result.status}`);
}
onEnd(result) {
console.log(`Testing completed with ${result.status}`);
}
}
Conclusion
Playwright offers a robust, efficient approach to API testing that can significantly improve your testing workflow. By following the patterns and practices outlined in this guide, you can build reliable, maintainable API tests that catch issues early and provide confidence in your applications.
Remember that effective API testing is an ongoing process. Regular review and updates of your test suite ensure it remains valuable as your application evolves.
Additional Resources
Have questions or suggestions? Join our community discussion below !
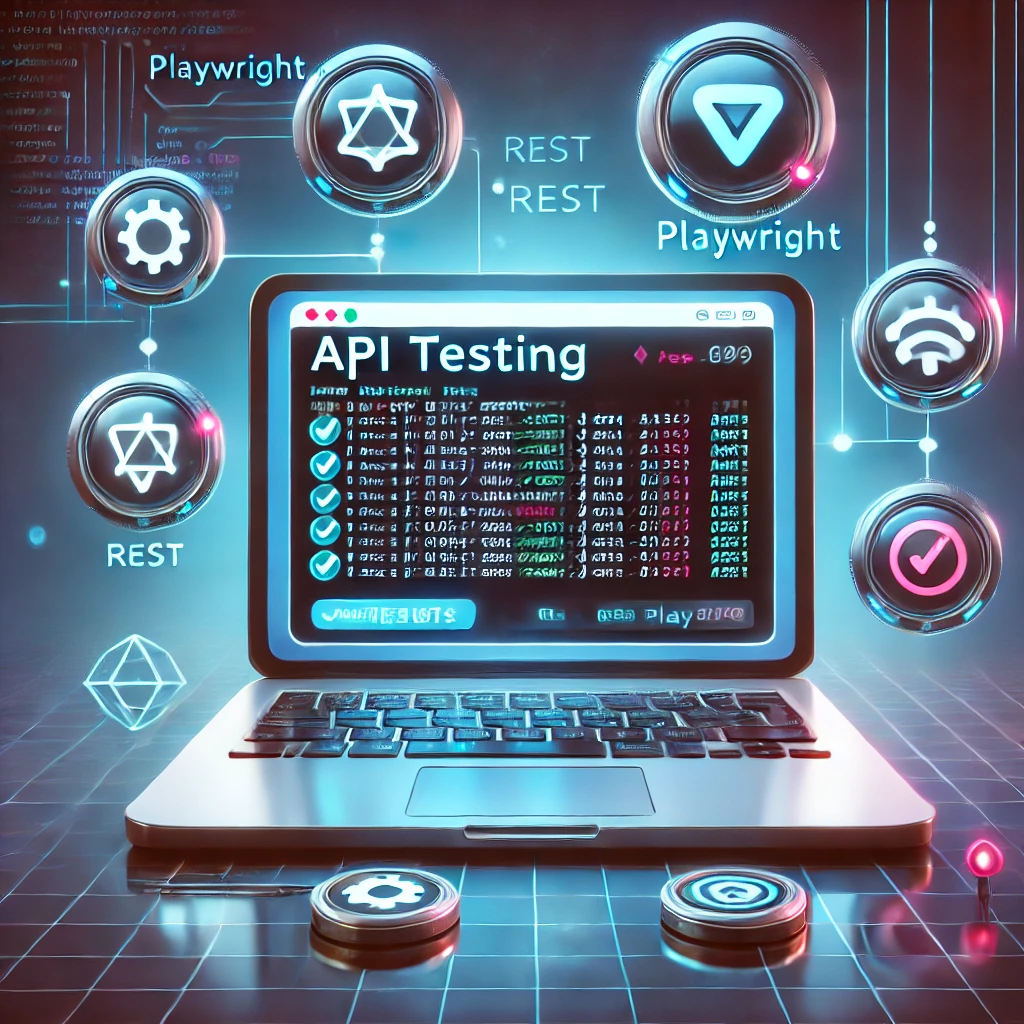